Stripe Payment Gateway — new Payment Method
When it comes to Magento 2, being the most popular Ecommerce platform, it provides many default payment methods. However sometimes you may need to create a custom payment method in Magento 2 store to integrate with your choice of payment gateway if it’s not already available.
In this tutorial, I will create a new payment method with stripe payment gateway step by step
Create a new Module Jeff_Stripe
module.xml file
#app/code/Jeff/Stripe/etc/module.xml
registration.php file
#app/code/Jeff/Stripe/registraion.php
composer.json file
{ "name": "jeff/module-stripe", "description" : "Jeff Stripe Payment Gateway", "require": { "php" : "~5.5.0|~5.6.0|~7.0.0" }, "type": "magento2-module", "version" : "0.0.1", "license" : [ "OSL-3.0", "AFL-3.0" ], "autoload" : { "files": [ "registration.php" ], "psr-4": { "Jeff\\Stripe\\": "" } } }
Backend configuration and default values
config.xml file for default values of some settings
#app/code/Jeff/Stripe/etc/config.xml 1 Jeff\Stripe\Model\Payment autorize_capture Jeff Stripe Payment Gateway AE,VI,MC,DI,JCB 0 1
system.xml file
#app/code/Jeff/Stripe/etc/adminhtml/system.xml Magento\Config\Model\Config\Source\Yesno Magento\Config\Model\Config\Backend\Encrypted Test/Live Secret Key Jeff\Stripe\Model\Source\Cctype Magento\Payment\Model\Config\Source\Allspecificcountries Magento\Directory\Model\Config\Source\Country $1 is the minimum amount allowed by Stripe Payment If customer tries to checkout with basket value greater than the maximum allowed they will be prevented from completing the order.
di.xml file to modify the arguments for the constructor of class Magento\Payment\Model\CcGenericConfigProvider
#app/code/Jeff/Stripe/etc/di.xml - Jeff\Stripe\Model\Payment::METHOD_CODE
creating source class Jeff\Stripe\Model\Source\Cctype
#app/code/Jeff/Stripe/Model/Source/Cctype.php
At this point, we finished the backend creation. After clearing cache and reload, you will find the new Payment method at backend as following image
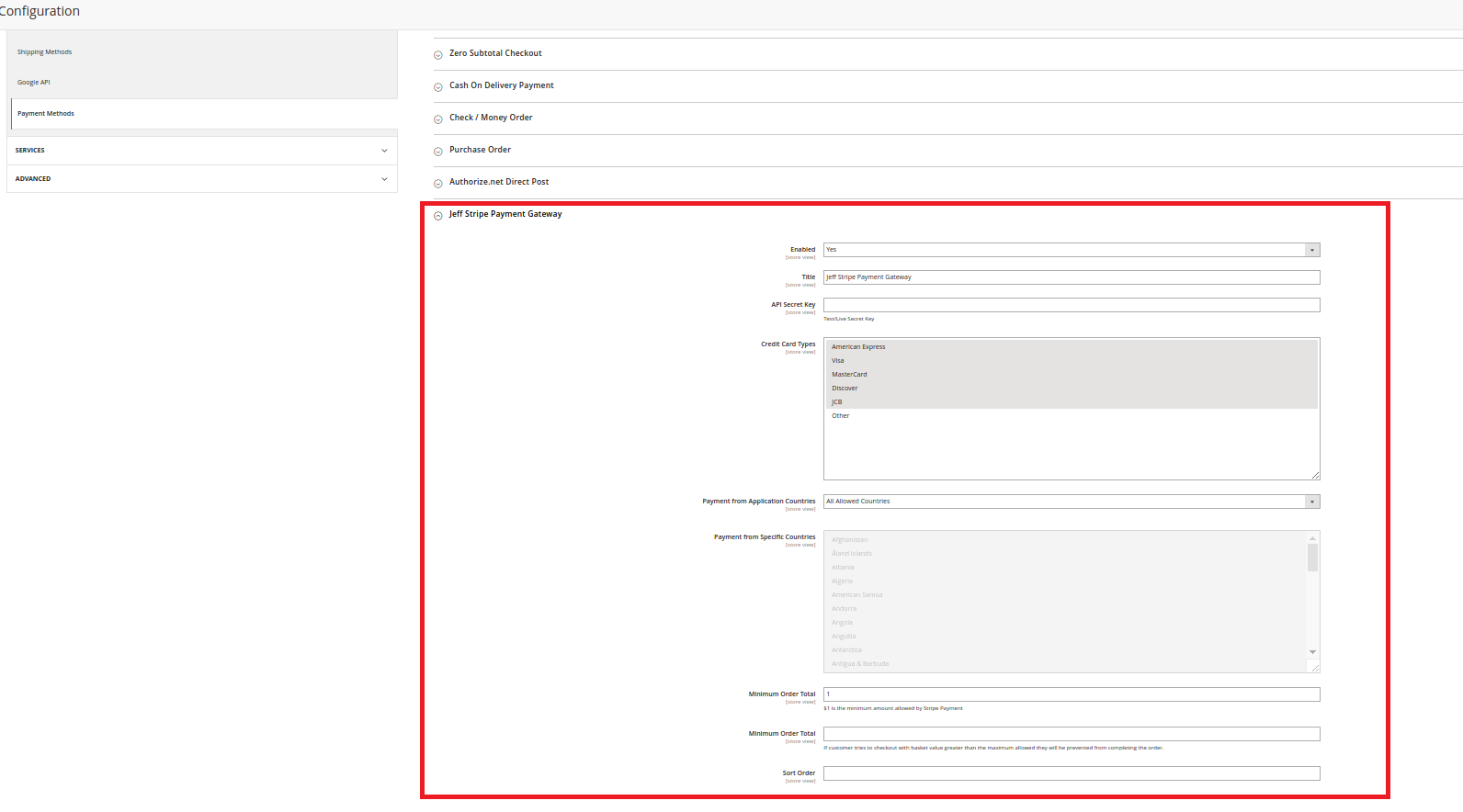
The Model adapter for the frontend payment method
Stripe Payment class
#app/code/Jeff/Stripe/Model/Payment.php _code = 'stripe'; $this->_stripe = $stripe; $this->_stripe->setApiKey($this->getConfigData('api_key')); $this->_minOrderTotal = $this->getConfigData('min_order_total'); } public function canUseForCurrency($currencyCode) { if (!in_array($currencyCode, $this->_supportedCurrencyCodes)) { return false; } return true; } public function capture(\Magento\Payment\Model\InfoInterface $payment, $amount) { $order = $payment->getOrder(); $billing = $order->getBillingAddress(); try{ $charge = \Stripe\Charge::create(array( 'amount' => $amount*100, 'currency' => strtolower($order->getBaseCurrencyCode()), 'card' => array( 'number' => $payment->getCcNumber(), 'exp_month' => sprintf('%02d',$payment->getCcExpMonth()), 'exp_year' => $payment->getCcExpYear(), 'cvc' => $payment->getCcCid(), 'name' => $billing->getName(), 'address_line1' => $billing->getStreet(1), 'address_line2' => $billing->getStreet(2), 'address_zip' => $billing->getPostcode(), 'address_state' => $billing->getRegion(), 'address_country' => $billing->getCountry(), ), 'description' => sprintf('#%s, %s', $order->getIncrementId(), $order->getCustomerEmail()) )); $payment->setTransactionId($charge->id)->setIsTransactionClosed(0); return $this; }catch (\Exception $e){ $this->debugData(['exception' => $e->getMessage()]); throw new \Magento\Framework\Validator\Exception(__('Payment capturing error.')); } } public function refund(\Magento\Payment\Model\InfoInterface $payment, $amount) { $transactionId = $payment->getParentTransactionId(); try { \Stripe\Charge::retrieve($transactionId)->refund(); } catch (\Exception $e) { $this->debugData(['exception' => $e->getMessage()]); throw new \Magento\Framework\Validator\Exception(__('Payment refunding error.')); } $payment ->setTransactionId($transactionId . '-' . \Magento\Sales\Model\Order\Payment\Transaction::TYPE_REFUND) ->setParentTransactionId($transactionId) ->setIsTransactionClosed(1) ->setShouldCloseParentTransaction(1); return $this; } public function isAvailable(\Magento\Quote\Api\Data\CartInterface $quote = null){ $this->_minOrderTotal = $this->getConfigData('min_order_total'); if($quote && $quote->getBaseGrandTotal() < $this->_minOrderTotal) { return false; } return $this->getConfigData('api_key', ($quote ? $quote->getStoreId() : null)) && parent::isAvailable($quote); } }
Knockout view model file: stripepayments.js
#app/code/Jeff/Stripe/view/frontend/web/js/view/payment/stripepayments.js define( [ 'uiComponent', 'Magento_Checkout/js/model/payment/renderer-list' ], function ( Component, rendererList ) { 'use strict'; rendererList.push( { type: 'stripe', component: 'Jeff_Stripe/js/view/payment/method-renderer/stripemethod' } ); /** Add view logic here if needed */ return Component.extend({}); } );
Stripe knockout model: stripemethod.js
#app/code/Jeff/Stripe/view/frontend/web/js/view/payment/method-renderer/stripemethod.js define( [ 'Magento_Payment/js/view/payment/cc-form', 'jquery', 'Magento_Checkout/js/action/place-order', 'Magento_Checkout/js/model/full-screen-loader', 'Magento_Checkout/js/model/payment/additional-validators', 'Magento_Payment/js/model/credit-card-validation/validator' ], function (Component, $) { 'use strict'; return Component.extend({ defaults: { template: 'Jeff_Stripe/payment/stripe1' }, getCode: function() { return 'stripe'; }, isActive: function() { return true; }, validate: function() { var $form = $('#' + this.getCode() + '-form'); return $form.validation() && $form.validation('isValid'); } }); } );
The view file for the knockout view model
#app/code/Jeff/Stripe/view/frontend/web/template/payment/stripe1.html
layout update file for checkout_index_index handle
# app/code/Jeff/Stripe/view/frontend/layout/checkout_index_index - uiComponent
- Jeff_Stripe/js/view/payment/stripepayments
- true
Install Stripe-php module with composer and enable our new payment method with following CLI commands
composer require stripe/stripe-php php bin/magento setup:upgrade php bin/magento setup:static-content:deploy php bin/magento cache:clean
Following picture demonstrates our new payment method is working
I hope my tutorial will help you create your own payment method easily
You could download the source code from GitHub.